Blog
Optimize your Frontend Engineering with ReactJS
June 21, 2023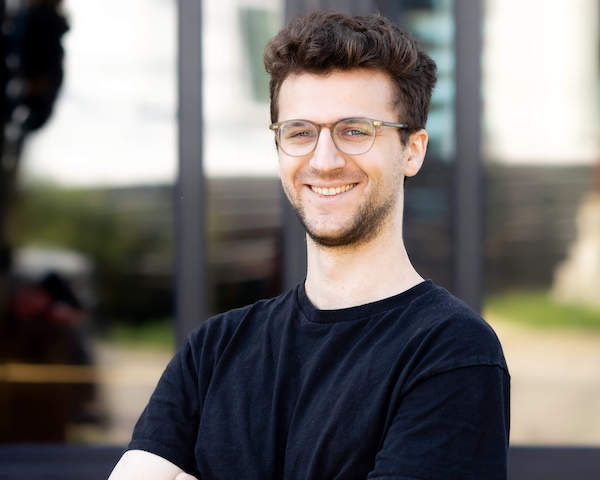
Theodore Schreiber
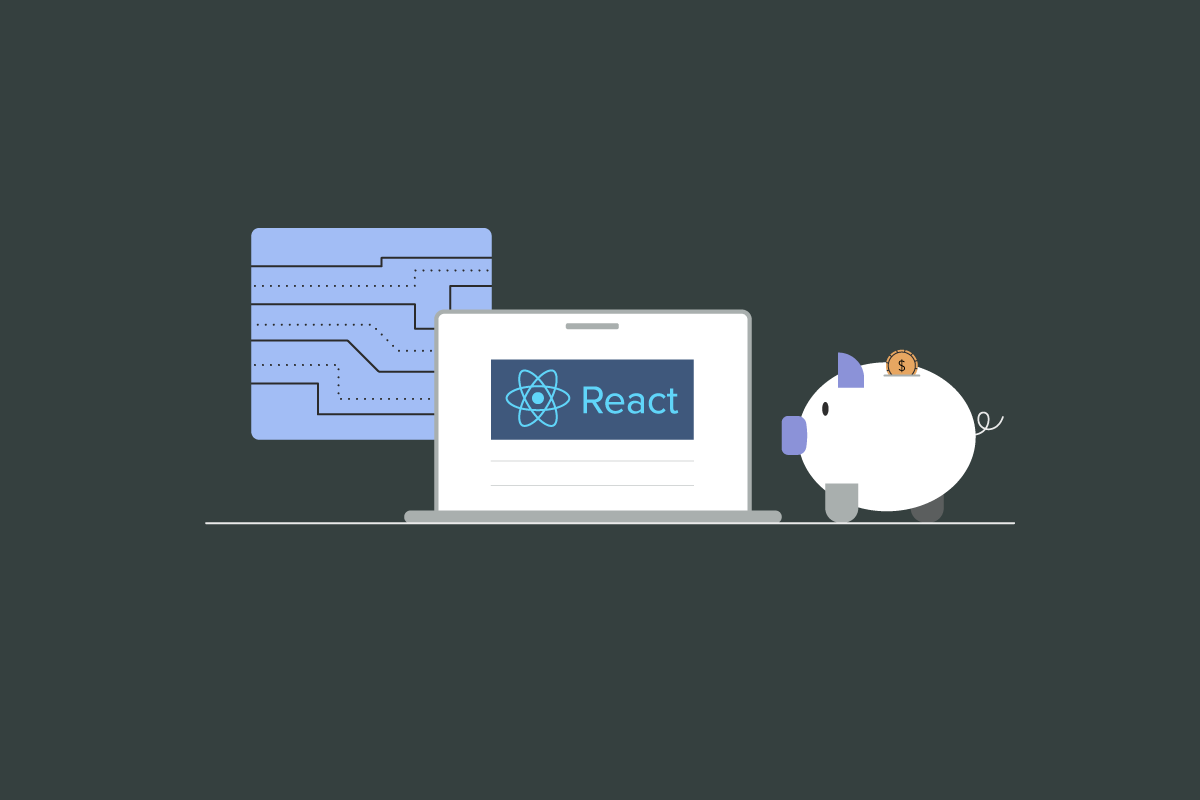
Every application, whether it is a desktop web application or a mobile app, needs a user interface (UI). From a user’s point of view, UIs should be fast to respond to changes, intuitive to understand, and visually appealing. From a developer perspective, frameworks and libraries that are used to develop UIs should ideally be easy to learn, popular, well-documented, maintainable, scalable, and lend to code which is easy to read.
At Link Money, the library we use for web development is ReactJS. We find it is highly performant for technical reasons detailed later in this post, easy to debug issues due to the massive community using it, and provides a template for code components which is both easy to read and reuse.
ReactJS’s Place in Javascript History
To understand the place and purpose of ReactJS, we will quickly look at the history of Javascript and some of its popular frameworks. Javascript was invented in the early 1990s to provide user interactivity in web pages. In its initial form, it was extremely barebones. While it was versatile and supported multiple programming patterns such as object-oriented, imperative, and functional, it had significant issues with performance, developer experience, and browser compatibility.
AJAX web development techniques came around in 1996 to address performance issues by providing the ability to load data asynchronously, thus decoupling the loading of data from the code providing the view to the end user. This allowed websites to update content without having to reload the entire page, a major improvement in user experience.
jQuery was invented in 2006, which among other features provided abstractions to browser-specific features that allowed developers to write code that would work across different browsers. This greatly improved the ease of web development and addressed bugs that users would face when websites were not developed with these browser eccentricities in mind. jQuery sits somewhere between functional and object-oriented programming.
Angular then took the developer world by storm in 2010 by providing a superior developer experience due to its intuitive workflow and exposing features such as data binding and dependency injection, using the model-view-controller design paradigm. Angular quickly became the most widely used Javascript framework for these reasons.
However, developers were still unsatisfied with the state of affairs in frontend development. As is the case throughout the history of software engineering, there was room for high-level abstractions, in this case for performance, scalability, and ease of development.
Enter Web Development with ReactJS
React was invented in 2011 by Jordan Walke, a software developer at Meta (formerly Facebook). It quickly came into competition with Angular as developers warred over which was the superior way to develop with Javascript. React eventually became the most popular Javascript technology and is now used by the likes of Instagram, Netflix, Imgur, Airbnb, and many more super successful companies.
Key Features of ReactJS: what makes ReactJS so popular?
Reactive Programming Paradigm
ReactJS uses a programming paradigm called “reactive programming”, which has many distinctive features and benefits over the aforementioned object-oriented, imperative, and functional programming patterns. While traditional coding uses steps which are executed sequentially, reactive programming receives asynchronous events using listeners whose order of execution depends on the order of those events. This creates programs where the order in which lines of code are written often does not matter. When applied to user interfaces, this means that code which responds or “reacts” to user events such as clicking and typing can be concise, easy to both write and understand, and abstract.
Declarative code
In alignment with the expectations of high-level abstractions, code written in these high-level frameworks should describe what the developer intends to happen, not how it is implemented. This is captured in the declarative vs imperative paradigm. As ReactJS uses the reactive programming paradigm, it is a declarative Javascript library. The consequence of this is that developers can update the state of ReactJS components by a simple function call and express listeners to changes in state that can have any desired functionality such as updating the UI or triggering an API call. ReactJS developers reap the benefits of the declarative library by not having to worry about the implementation logic under the hood, providing a comfortable developer experience, rapid development time, and easily readable code.
Reusable Components
Modular building blocks are a concept which should be familiar to most if not all developers. Functions, classes, and modules all allow developers to reuse code, facilitating scalability and giving means to develop third-party libraries. ReactJS components give developers units of code which have an internal state and can be composed of other components. This allows for ample expressive and state variable naming, lending naturally to scalable, readable, self-documenting code. Components can be imported from other libraries or created as custom components.
Virtual DOM
HTML content is organized in the browser as a tree known as the Document Object Model (DOM). In order to achieve ideal performance, ReactJS creates a virtual representation of the DOM. When an action triggers a change in the UI, ReactJS computes the changes on this virtual DOM while leveraging its reusable components and makes the minimum possible changes to the real DOM, creating a seamlessly performant user experience. In the age of ADHD users, quick updates to UIs are an attractive feature for products to possess.
Popularity
Increased popularity of a language, framework, or library creates a feedback loop which makes it more and more popular - this is known as the network effect. Since ReactJS has very strong community support, it is easy to find other developers who have expertise in the technology, as well as written and video online resources to learn about its core features and troubleshoot issues. This gives ReactJS an edge over potentially superior but less used technologies.
Evolution of ReactJS
ReactJS is constantly being updated - a feature of the library which has both pros and cons. While new features allow for enhancements and improvements over older features, it requires extra effort from developers to keep up to date with changes which are not always not well documented.
The most significant change in ReactJS’s history has been the switch from class components to functional components. This was a simplification of syntax creating more readable code, and allowed for simpler development around the lifecycle of components. Functional components are simply Javascript functions which take the component props as an argument and return JSX. Hooks came around with functional components in order to help manage state and component lifecycle - hooks can be thought of simply as function calls within a functional component that allow you to use features of ReactJS, third-party tools, and your own custom code.
Another current step in the progression of ReactJS is the integration of server components. This is a new feature which allows developers to render their components in the server, creating separation of responsibilities between the client and server which results in cleaner code. Any non-interactive components can be moved to the server such as data fetching, logic related to authentication or authorization, and pre-processing of data. The benefits of this include a much faster user experience since rendering takes place in your server, smaller bundle size resulting in better performance, and slightly better security as you can relegate sensitive information such as environment variables to the server. However, since the code is not running in the browser, there are some features which are unavailable to server components such as common ReactJS hooks (useState, useEffect) and typical browser features (localStorage, window).
What does ReactJS not do?
ReactJS only concerns itself with the UI, in particular with the relationship between components and the DOM, applications using ReactJS will have to use additional libraries for features such as styling (e.g. styled-components), API calls (e.g. Axios), and app-wide state management (e.g. react-redux). This flexibility comes with pros and cons.
While developers benefit from customization by using their preferred libraries for this functionality, there is a lack of uniformity across ReactJS applications that can make it difficult for ReactJS developers to understand ReactJS apps with unfamiliar libraries. Furthermore, the increase of external dependencies means that there can be compatibility issues when those dependencies are updated. Alternatives to ReactJS such as Angular and Vue are more prescriptive with dependencies and therefore while they lack customization, they are more organized and consistent.
Another drawback of ReactJS is that it uses a unique wrapper over HTML called JSX in order to combine HTML and Javascript into one syntax. This increases the learning curve for developers to get started writing ReactJS, though JSX is not considered to have other significant downsides.
Will anything overtake ReactJS in the future?
As of now, ReactJS remains the most popular Javascript library or framework to develop frontend applications. However, some alternatives are available which may or may not increase in popularity in the coming years. Even if you choose to only develop with ReactJS, it is wise to be aware of these technologies in the event that ReactJS eventually is surpassed in overall quality.
Vue JS
Similarly to ReactJS, Vue JS is versatile, readable, and performant. It contains HTML, CSS and Javascript in a single page which creates readable and understandable code. As mentioned in the previous section, Vue includes more built-in functionality than ReactJS such as app-wide state management, however it suffers from having less third-party support tools. It also includes the benefits of performance by virtualizing the DOM, and scalability through reusable components. The major drawback of Vue is its small developer community in comparison with ReactJS, making it more difficult to learn, troubleshoot issues, and scale to large enterprise-grade applications. If and when its ecosystem grows, Vue could challenge ReactJS as the most popular Javascript framework.
Svelte
Svelte is highly regarded in today’s landscape of Javascript framework. It performs slightly better than ReactJS in practice. It is easily scalable due to its use of modular components. There is a shorter learning curve due to Svelte’s simple methodology of using straightforward HTML, CSS, and Javascript, tools already familiar to front end developers. While it is currently less popular than ReactJS, it has a slightly higher developer satisfaction rate. Its popularity is growing and is certainly a framework to look out for in the future.
ReactJS’s Little Brother - Mobile App Development with React Native
While ReactJS is used for web applications, React Native is used for mobile app development of applications that are cross-platform - that is, they can run on both Android and iOS devices. In addition to developing our web services with ReactJS, Link Money provides a React Native SDK for merchants to more easily integrate with our services.
React Native was developed at a hackathon with the intent of giving developers the means to develop mobile apps without having to acquire special knowledge of the eccentricities of each operating system, and the ability to leverage their experience with ReactJS, HTML, CSS, and Javascript to write very similar code. This is possible because ReactJS and React Native share similar syntax and design patterns. This was a similar concept to how jQuery gave developers abstractions to browser-specific behaviors which improved the developer experience, shortened the learning curve, and removed many instances of OS-specific bugs.
Pros of React Native
Further benefits of the similarities between React Native and ReactJS include that many popular libraries used in conjunction with ReactJS have an equivalent version for React Native including Axios, Tailwind CSS, and Bootstrap CSS. The already stated benefits of ReactJS such as performance through the virtual DOM, scalability through modular components, and popularity all apply to React Native. ReactJS developers should strongly consider React Native as a candidate when creating mobile applications.
Cons of React Native
However, like ReactJS, React Native is not perfect. The simplification of providing device-agnostic code comes with the cost that several features are slightly inferior in comparison with native applications. These include performance, development of complex interfaces, and debugging. Furthermore, updating versions of React Native has in practice been regarded as more complex than it should be. The tradeoff between these factors and the ease of development should be considered when deciding whether to develop with React Native.
Summary
In conclusion, ReactJS and React Native will provide your frontend engineering team with an easy-to-learn, performant, and scalable way to create website and mobile applications. It is important to understand the pros and cons of the flexibility that it provides when deciding whether to use ReactJS and React Native. In the future, watch out for VueJS and Svelte to be key players in the frontend framework space as they attempt to overcome the Goliath of ReactJS.